정적컨텐츠
값의 변화 없이 저장된 파일을 웹브라우저에 띄운다(그냥 내린다)
// 컨트롤러
@GetMapping("hello")
public String hello(Model model){
model.addAttribute("data", "hello!!");
return "hello";
}
※ 컨트롤러에서 data의 속성값 hello!! 을 직접 받고 html로 전달되어 화면에 나타남
// hello.html
<!DOCTYPE HTML>
<!--타임리프 템플릿 엔진, 타임리프 문법사용 가능-->
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Hello</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8"/>
</head>
<body>
<p th:text="'안녕하세요. '+ ${data}" >정적페이지 반환</p>
</body>
</html>
※ th:text 에서 입력한 값으로 바뀌므로 p태그 사이의 입력값은 무시해도 좋음
MVC와 템플릿엔진
외부(웹)에서 파라미터를 받아 컨트롤러에 전달되고 Model에 담은 후 템플릿으로 넘어가면 값이 치환된다
뷰를 통해 렌더링 후 변환된 html 을 웹브라우저에 띄운다
@GetMapping("hello-mvc")
public String helloMvc(@RequestParam("name") String n, Model model){
model.addAttribute("name", n);
return "hello-template";
}
// hello-template.html
<html xmlns:th="http://www.thymeleaf.org">
<body>
<p th:text="'hello '+ ${name}" >hello! empty</p>
</body>
</html>
※ 템플릿엔진으로 p태그 사이의 값이 th:text 의 값으로 치환된다
※ 웹에서 파라미터값을 넘겨줘야 함
API
html 을 통하지 않고 그대로 데이터를 화면에 나타낸다
맛보기)
더보기

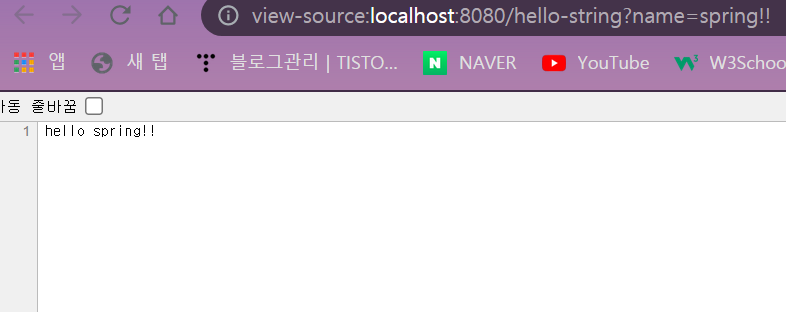
소스보기
@GetMapping("hello-string")
@ResponseBody
public String helloString(@RequestParam("name") String n){
return "hello " + n;
}
※ ResponseBody: http 통신 프로토콜 body부에 데이터를 직접 넣어주겠다

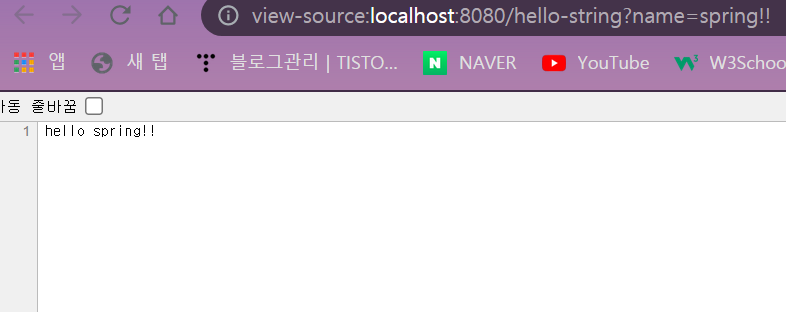
※ html 을 통하지 않았다
본격 api)
객체를 넘겨주었다
ResponseBody 가 있고 객체를 반환한다면 기존의 HttpMessageConverter의 동작으로 JsonConverter가 동작해
Json방식('키:값' 의 쌍으로 이루어진 데이터구조)으로 웹브라우저에 띄운다
@GetMapping("hello-api")
@ResponseBody
public Hello helloApi(@RequestParam("name") String n){
Hello hello = new Hello();
hello.setName(n);
return hello;
}
static class Hello {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
api 정리)
HTTP의 body부에 문자내용은 직접 반환한다
객체라면 viewResolver 대신에 HttpMessageConverter 가 동작, 기본 문자는 StringHttpMessageConverter 가 동작하고
기본 객체는 MappingJackson2HttpMessageConverter가 동작한다
'Spring' 카테고리의 다른 글
[Spring] 싱글톤 컨테이너와 @Configuration (0) | 2021.11.28 |
---|---|
[Spring] @Configuration, @Bean 이해하기 (0) | 2021.11.26 |
[Spring] DI : Dependency Injection (0) | 2021.08.28 |
[Spring] Optional <T> (0) | 2021.08.26 |
[Spring] Windows에서 cmd로 빌드&제거 (feat. IntelliJ) (0) | 2021.08.22 |